In this challenge, we are presented with an executable file unlike the first challenge where we also had access to the source code. Just like any other programming / reversing challenge, this one could have been possibly solved by two ways (I could think of two, there may be more). Following is my write-up for the challenge.
Challenge 2 – Garbage
On the challenge screen, we are presented with a 7z file which after being unzipped gives us two files – An executable named garbage.exe and a text file named Message.txt.
The message file describes the challenge. As per the message, it is a corrupted binary extracted via extreme forensic techniques.

Alright, so as per the description, it is possibly a corrupted executable file which needs to be recovered and then reverse engineered. Let’s understand the file by taking a quick look in CFF explorer. Looking at the executable in CFF explorer, the following points were noted:
- The file is PE32
- Packed with UPX
- The Import Directory’s RVA is invalid
- It is missing the Imports directory and the relocation directory
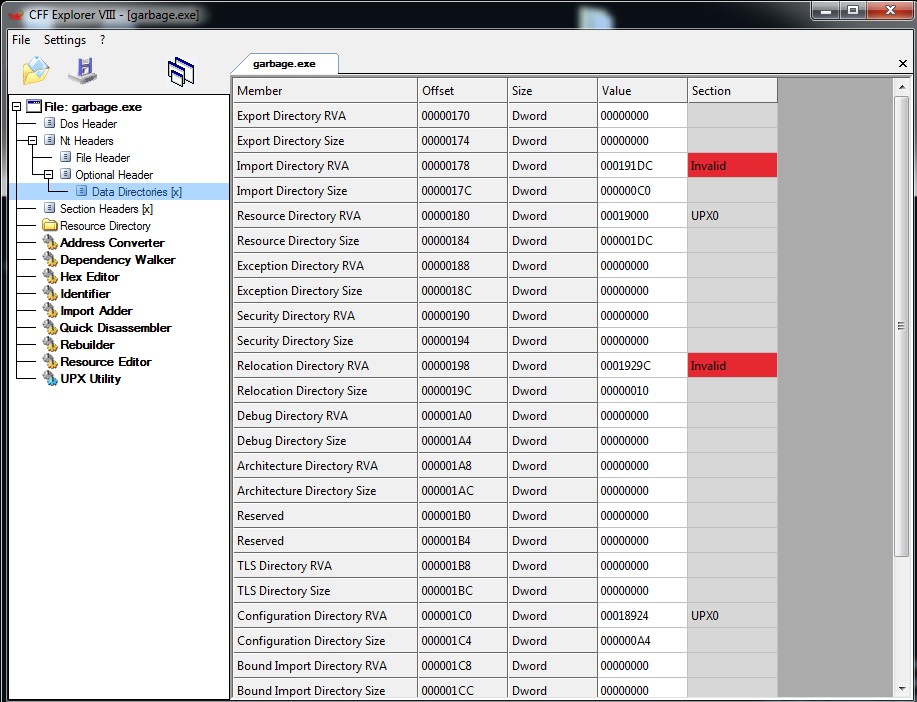
Now that we know the file is packed with UPX, we can go ahead and unpack it. Quick UPX 101s before we dive further:
- UPX doesn’t involve any kind of encryption, in that it only compresses the file
- Works as a “stub-payload” architecture
- It is Open source
Let’s unpack!
Unpacking binaries packed with UPX is simple. We can do it with UPX’s command line utility simply by passing the -d parameter and our executable as a parameter. Alternatively, we can also use CFF explorer’s in-built UPX unpacker. Let’s attempt to unpack our executable using the same.
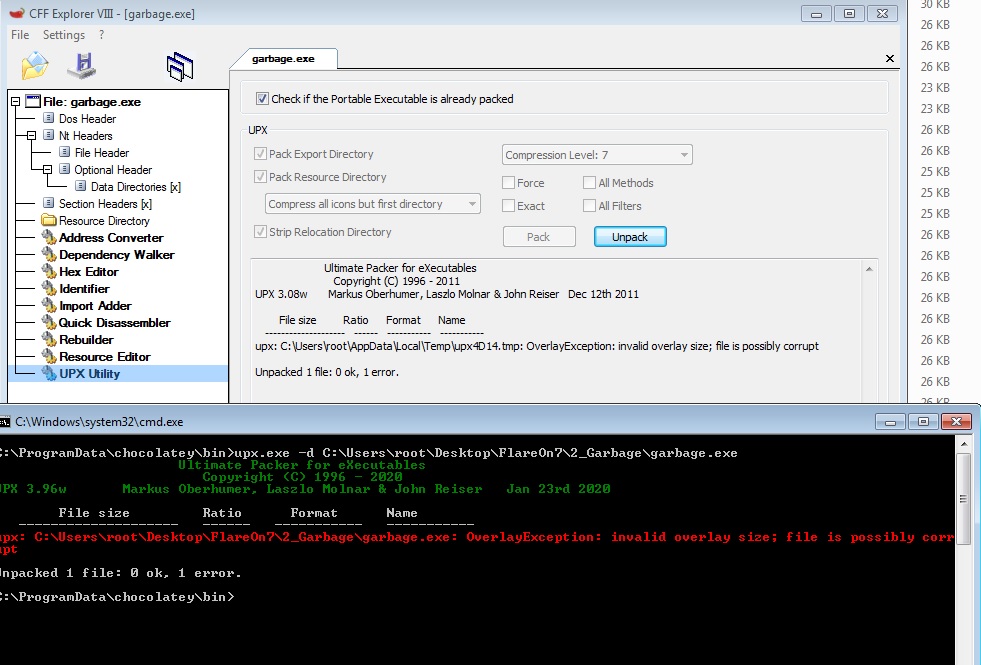
Awkward. 😀
Evidently, both the CFF explorer’s inbuilt utility and the standard UPX utility is unable to unpack our executable. Luckily for us, UPX is open sourced and hence we can pin-point to the exact line of code where this error is prompted. Doing a Github code search for our error string, the following part of UPX code was identified:
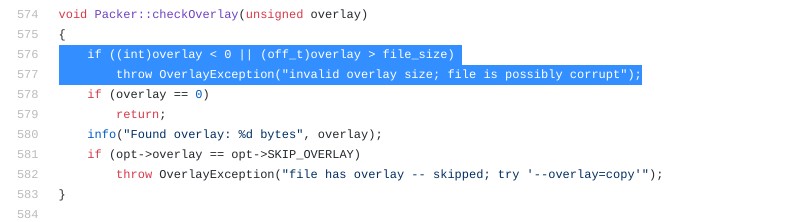
Which basically means that the file size is actually different than what the headers say. Going back to CFF explorer and taking a look at the File Size and the PE Size, it becomes evident that the file lacks ~750 bytes. Further, taking a look at the file in a Hex editor, it becomes clear that the bottom of the file is truncated which apparently looks like the manifest. To overcome this issue, we can take a standard executable, pack it with UPX and append the bottom part from a HEX editor of the newly packed file to our executable under analysis. I had recently created a custom tool for one of our red team engagements which involved messing around with file pointers and appending data to files (More on that later). That turned out to be helpful in formulating the above solution and also I had an executable handy to pack it with UPX.

Alright, now that we have a new file with appended data, UPX should be able to unpack it.
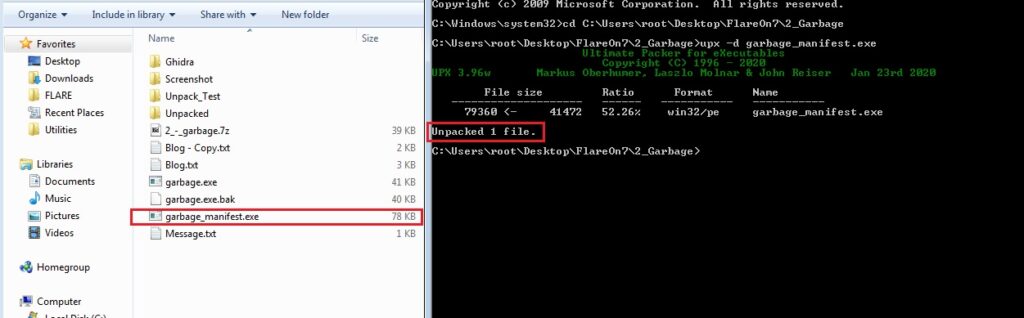
And there we go, as seen above, UPX successfully unpacked the file. A normal UPX unpacked file should ideally be able to run as expected. However, in our case, the Import directory is invalid thus executing the unpacked file won’t fetch any results. To confirm, I attempted to execute the file and was presented with the following error:
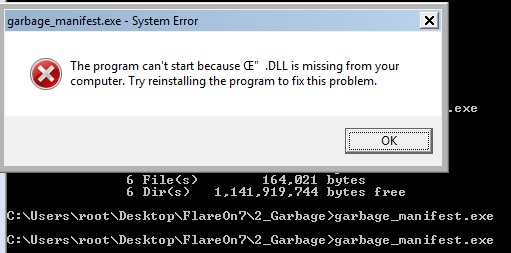
This was a dead end of a sorts but after spending some time researching on ways to overcome the issue, I was able to find the following two ways:
- Re-construct the Import Address Table
- Analyse the file statically
I wasn’t much confident on the re-construction part due to the nitty-gritty of address space arithmetic. Hence I went ahead with option two – analysing the file statically. And also because I wanted to put some of my newly acquired RE skills to test.
Obviously, we wouldn’t have got the flag just by using Strings on the binary but like we do it in every CTF or even malware analysis for that matter, I did it. I prefer to use Floss to identify strings for its versatile string identification functionalities. Gives a bit more than the vanilla strings.exe.
Below is the screenshot from Floss’s output:

Nothing interesting apart from the two unusually long strings on the stack. Let’s park these strings for now.
Reversing
Now that we have an unpacked binary, we can go ahead and disassemble it for further analysis. I used Ghidra to reverse the executable. Loading up the binary in Ghidra, and looking at the defined strings, we can see the same two strings as identified by Floss along with an easter egg referencing COVID-19.
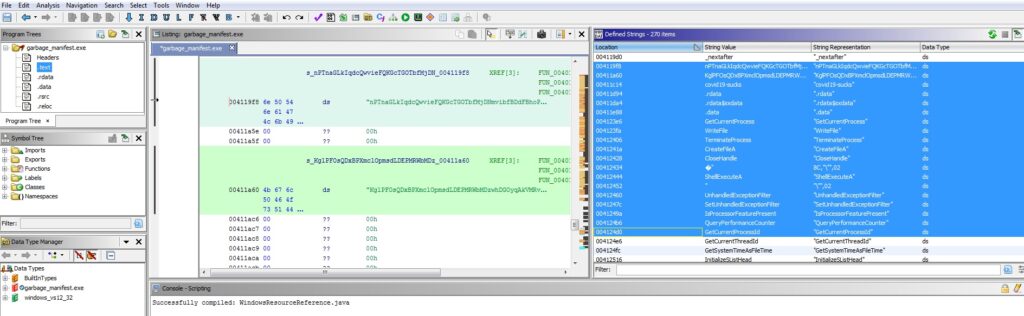
From the looks of it, the executable appears to write a file but there’s not much we can conclude with a broken import address table. I spent a lot of time exploring around the disassembly aimlessly. For a new reverser, the disassembly can get quite overwhelming in the beginning. I decided to dig deeper on the two long strings that we identified and went ahead to find out the references to the strings.

Looking at the references, we can see that after both the strings have been set-up, the function FUN_00401000 is called. Before diving into FUN_00401000 let’s take a look at the call graph.
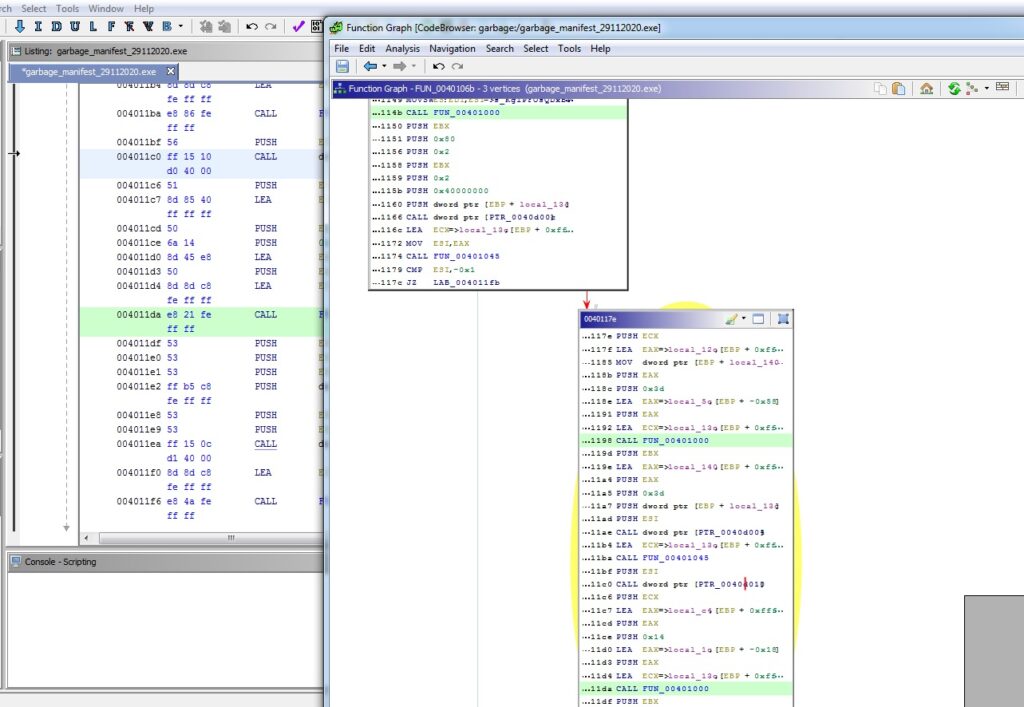
We can see that the function is called three times and after each call, we can see a unique function call. Inspecting the function call after the first instance of FUN_00401000, we can see that the call is being made to CreateFileA. Similarly, after the second instance, the call is made to WriteFileA then to CloseHandle and lastly to ShellExecutaA.
Evidently, a file is being created then something is being written in the file and lastly the file is executed with ShellExecuteA.
If we see the chronology of the function calls, it appears that the function – FUN_00401000 is called before the file is written and then the second time before the content of the file is written inside the file and lastly when the filename is called via ShellExecuteA to execute the file.
Now that we have a fair understanding of the control flow, let’s go ahead and take a look at the disassembly of function – FUN_00401000 itself.
In the disassembly, we can see that a XOR operation is performed. Further, looking at the decompiled form of the disassembly it gets clear that the XOR operation is performed on parameter one with the third parameter while the count is less than parameter two.
Below is a slightly cleaner version of the decompiled form:
int * __thiscall FUN_00401000(void *this,int param_1,int param_2,int param_3)
{
uint counter;
counter = 0;
*(int *)this = param_1; // &local_1c = Encoded data
*(int *)((int)this + 4) = param_2; // Constant value (0x3d for instance 1)
*(int *)((int)this + 8) = param_3; // Long string
*(undefined4 *)((int)this + 0xc) = 0x66; //0x66 = Decimal 102
if (param_2 != 0) {
do {
//this->param_1[counter] = this->param_1[counter] ^ this->param_3[counter % 0x66]
param_1[counter] = param_1[counter] ^ param_3[counter % 0x66]
counter = counter + 1;
}
//while (counter < *(uint *)((int)this + 4));
while (counter < param_2);
}
return (int *)this;
}
Now let us try to figure out what parameters are being passed to the function. As per the standard calling convention, all the parameters are first pushed in the registers then the regisrters are pushed on the stack and lastly the function call is made. Keeping this in mind, I identified the parameters that are pushed on the stack for the function – FUN_00401000.
After identifying the paramters that are pushed, we can see that the following arguments are passed to our function:
- Instance 1 – FUN_00401000(0x2c332323, 0x3d, param_3)
- Instance 2 – FUN_00401000(0x3b020e38h, 0x14, param_3)
Wherein parameter one appears to be the encoded data which is spread across the code in the form of static variables and parameter three is nothing but one of the long strings that we identified in the beginning. Stitching our analysis together, we can port the function in a Python version as seen below:
def decode(encoded_data, limit, long_string):
counter = 0
store_result = ""
# if limit:
while (counter < limit):
temp = long_string[counter] ^ encoded_data[counter % 0x66]
store_result = store_result+chr(temp)
counter = counter + 1
print(store_result)
def main():
encoded_data_1 = bytes.fromhex("380E023B193B1B341B0C233E3308114239121E73")
encoded_data_2 = bytes.fromhex("2323332C0E3F64490A1E0A042316021A446608243211742C2A2D420F3E50640D5D041B1716360305342009086321240E151434581A29793A0000565854")
decode(encoded_data_2, 0x3d,b"nPTnaGLkIqdcQwvieFQKGcTGOTbfMjDNmvibfBDdFBhoPaBbtfQuuGWYomtqTFqvBSKdUMmciqKSGZaosWCSoZlcIlyQpOwkcAgw")
decode(encoded_data_1,0x14,b"KglPFOsQDxBPXmclOpmsdLDEPMRWbMDzwhDGOyqAkVMRvnBeIkpZIhFznwVylfjrkqprBPAdPuaiVoVugQAlyOQQtxBNsTdPZgDH")
if __name__ == '__main__':
main()
And executing the above code should result in our flag!

SUCCESS

References: